Ok, the short answer to that question is easy, just assign it directly. That’s it, and this is the shortest blog post ever. But actually that’s not the point, I wanted to make. There are a bunch of methods to assign a class-property with a specific value, but how do they differ, when it comes to performance. Even though I can guess, that Reflection is probably very expensive, I have no real feeling to what degree. My measurements also came up with a surprise, I didn’t expect when I started this out.
The setting
For me, it’s very interesting to investigate that on .NET Standard with C# in UWP, because we are currently developing an App with Xamarin.Forms. Please keep in mind, that the results may differ on .NET Core or other targets, but I think there will be no dramatic difference.
We will generate a TestClass with a property Sum, afterwards, we will read the value, add an iteration variable to it, and write it back to the property. Let’s do that more than 10000 times, so we get a good feeling for the performance.
What possibilities we have?
Let’s do some brainstorming on what kind of methods we have to read and assign a value to a property.
- Direct assignment
obj.Sum = obj.Sum + i
- Reflection
var type = typeof(TestClass);
var sumProp = type.GetProperty("Sum");
var val = (int)sumProp.GetValue(obj);
sumProp.SetValue(obj, val + i);
- Function
var func = (Action)((TestClass o, int n) => { o.Sum += n; });
func(obj, i);
- Dynamic
dynamic objDyn = new TestClass();
objDyn.Sum = i + objDyn.Sum;
Let’s start the test
First of all, we try to find out how long the first call takes. I assume, that the first access of a property takes longest. Afterwards it’s probably way faster. But enough talk about what might happen, get it on:

The measurements are in 10-7 s. As we can see, the first calls with dynamic objects is very slow. All other methods are really close to each other. Is this just because of the Debug mode? How about compiling the code with .Net native? Maybe we can distinguish the methods better in Release Mode.

This seems to be a better solution. We can see, that direct assignments and function calls are very close, and take no time at all. The function call is a little bit slower, just because of the overhead of the function. Reflection is slightly better in .Net nativ (app. 50%) and dynamic calls are 95% faster in native build, than they are in debug mode.
But when we think about a real App, the first call isn’t very interesting. Just assume we have an App to manage our Customers. A customer object has 20 properties (name, street, zip code, etc.), we have about 5000 customers, and a page that shows all customers in a table. Our Model needs to be mapped to a ViewModel to show it on the screen. That gives us 100.000 assignments. I think this is a very realistic scenario, we can investigate on. (we will skip the first assignment call in our measurements)

What we can see, is that (as expected) function-calls and direct assignments take nearly the same time. Dynamic assignments are surprisingly fast, and reflection is very slow. But what does “slow” mean in that context? Have a look at the times measured:
- direct: 1,2 ms
- function: 2,0 ms
- dynamic: 7,6 ms
- reflection: 74 ms
We got so far, let’s have a look at the same setting with .Net native builds:
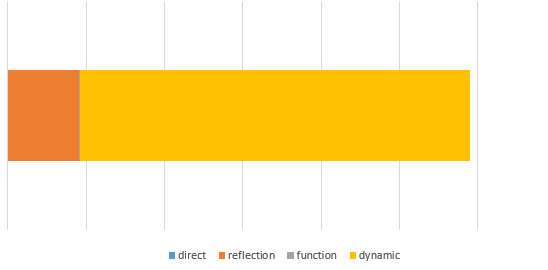
Where is direct and function? Both measurements are under 1ms, so there is a real boost. But there is a big surprise – What happened to dynamic????
- direct: 0,12 ms ( -90%)
- function: 0,3 ms ( -85%)
- reflection: 45 ms ( -39%)
- dynamic: 249 ms ( +337%)
I’ll investigate that issue on one of my future posts, if you have any ideas, how this could happen, please feel free to add a comment.
Conclusion
There is an eligible reason, why Microsoft introduced the dynamic objects. Dynamic objects are very common in scripting languages like PHP or JavaScript. When you use it to parse responses of a Rest-API or just want to read data from a Json in your own object structure. This is really neat because you don’t have to create an own class structure, that is only used as an intermediate layer.
I am very curious, so I also tried to use ExpandoObjects and in contrast to that a Dictionary<,>:
- ExpandoObject: 800 ms
- Dictionary<,>: 5 ms
So you should definitely try to use Dictionaries instead of any dynamic approach. It’s a little bit harder to code, but it seems to be worth it.
When it comes to reflection, you probably can not avoid it – it is also one of the big strength of C# and the .Net Framework, that makes it so flexible even when it’s still a strongly typed programming language. Although I will be careful in future developments, to find more conservative solutions, when possible.
Almost forgot… the winner is

direct assignment… I dare you knew that before 🙂